使用Entity Framework Core加入既有資料庫的模型
羅慧真 Anita Lo
- 精誠資訊/恆逸教育訓練中心-資深講師
- 技術分類:程式設計
Entity Framework Core 不像 .NET Framework 的 Entity Framework 可以透過圖形化工具產生既有資料庫的模型 (DbContext) ,但是可透過命令來建立模型,這一篇文章就是要說明如何建立既有資料庫的 EF Core 模型。
啟動Visual Studio 2019開發環境。從「File」-「New」-「Project」,在「Create a new project」對話盒中選擇 Console App (.NET Core) ,按下「Next」:
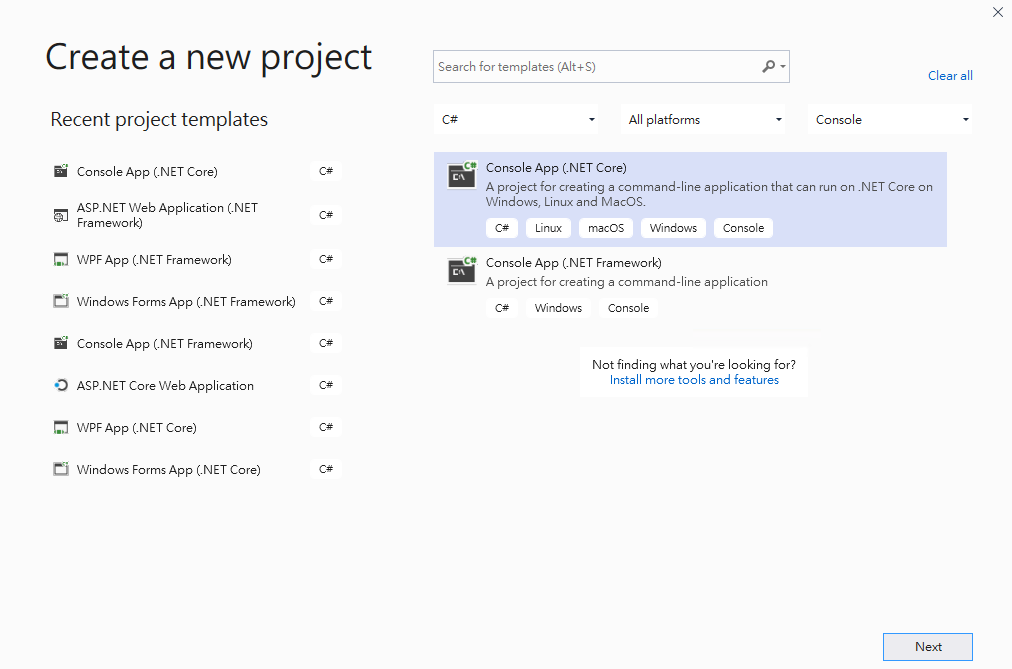
指定專案名稱之後按下「Create」。
使用 NuGet Package Manager 加入「Microsoft.EntityFrameworkCore.SqlServer」、「Microsoft.EntityFrameworkCore.Tools」。從選單選取「Tools」-「NuGet Package Manager」-「Manage NuGet Packages for solution」,在「Browse」頁裡分別搜尋「Microsoft.EntityFrameworkCore.SqlServer」、「Microsoft.EntityFrameworkCore.Tools」並安裝它們。在Soltuion Explorer 中可以看到安裝之後的套件。
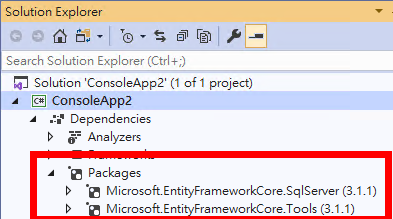
接著在PMC (Package Manager Console) 下Scaffold-DbContext指令,將建立既有資料庫的 EF Core 模型。從選單選取「Tools」 - 「NuGet Package Manager」 - 「Package Manager Console」,輸入以下指令:
Scaffold-DbContext "Server=.\SQLExpress;Database=Northwind;Trusted_Connection=True;" Microsoft.EntityFrameworkCore.SqlServer -OutputDir Models -Tables Employees,Orders
Scaffold-DbContext命令後面接的是資料庫連線字串 「"Server=.\SQLExpress;Database=Northwind;Trusted_Connection=True;" 」這將連接到本機SqlExpress伺服器裡頭的Northwind資料庫並以信任目前登入帳號登入資料庫。後面接著的是「Microsoft.EntityFrameworkCore.SqlServer」是資料庫提供者,-OutputDir參數指定要輸出的資料夾,-Tables 參數則是指定要產生模型的資料表名稱,若有多個使用逗點區隔。
執行完成在Soltuion Explorer 中看到EF Core 模型將被成功地建立Models 資料夾。
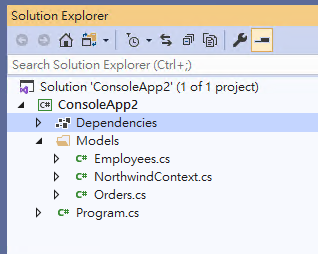
開啟 Models 資料夾中的 Employees.cs 你將會看到以下程式:
namespace ConsoleApp2.Models { public partial class Employees { public Employees() { InverseReportsToNavigation = new HashSet(); Orders = new HashSet (); } public int EmployeeId { get; set; } public string LastName { get; set; } public string FirstName { get; set; } public string Title { get; set; } public string TitleOfCourtesy { get; set; } public DateTime? BirthDate { get; set; } public DateTime? HireDate { get; set; } public string Address { get; set; } public string City { get; set; } public string Region { get; set; } public string PostalCode { get; set; } public string Country { get; set; } public string HomePhone { get; set; } public string Extension { get; set; } public byte[] Photo { get; set; } public string Notes { get; set; } public int? ReportsTo { get; set; } public string PhotoPath { get; set; } public virtual Employees ReportsToNavigation { get; set; } public virtual ICollection InverseReportsToNavigation { get; set; } public virtual ICollection Orders { get; set; } } }
Employees 類別仿照Northwind資料庫的Employees 資料表成為一個Entity類別。 開啟NorthwindContext.cs 檔案,內容如下:
public partial class NorthwindContext : DbContext { public NorthwindContext() { } public NorthwindContext(DbContextOptionsoptions) : base(options) { } public virtual DbSet Employees { get; set; } public virtual DbSet Orders { get; set; } protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder) { if (!optionsBuilder.IsConfigured) { #warning To protect potentially sensitive information in your connection string, you should move it out of source code. See http://go.microsoft.com/fwlink/?LinkId=723263 for guidance on storing connection strings.optionsBuilder.UseSqlServer("Server=.\\SQLExpress;Database=Northwind;Trusted_Connection=True;"); } } ~~~相關內容省略~~~ } partial void OnModelCreatingPartial(ModelBuilder modelBuilder); }
NorthwindContext類別繼承自 DbContext,並根據Scaffold-DbContext命令所傳遞的連線字串及資料表產生相對應內容。
接著可以透過NorthwindContext類別連接到資料庫,並存取資料內容。開啟Program.cs,在Main 程式區塊中建立NorthwindContext物件,使用foreach將Employees集合將資料內容列表出來,程式碼如下:
static void Main(string[] args) { using (var db = new NorthwindContext()) { foreach (var emp in db.Employees) { Console.WriteLine($"{emp.EmployeeId} - {emp.FirstName},{emp.LastName} - {emp.Title}"); } } }
執行結果如下:
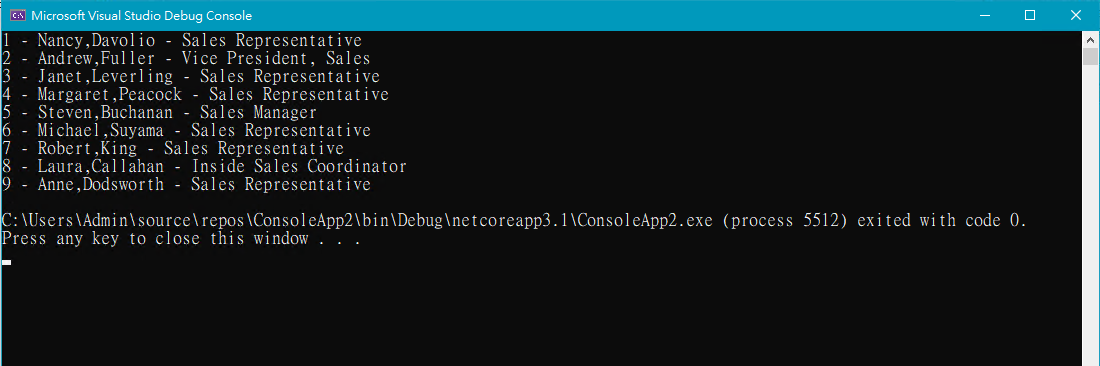